Cool one-liner solutions to interesting JavaScript problems.
1. Baffling shuffling magic: Arrays
Everyone always talks about shuffling arrays with sort()
and Math.random() - 0.5
…
But have you met my friend? 👇
const shuffleArray = (arr) =>
[...Array(arr.length)]
.map((_, i) => Math.floor(Math.random() * (i + 1)))
.reduce(
(shuffled, r, i) =>
shuffled.map((num, j) =>
j === i ? shuffled[r] : j === r ? shuffled[i] : num
),
arr
);
// [ 2, 4, 1, 3, 5 ] (varies)
console.log(shuffleArray([1, 2, 3, 4, 5]));
And isn’t it cool how the data just flows freely from input to output? No stops for intermediary values along the way👍
2. A need for belonging: Group array by ID
const groupBy = (arr, groupFn) =>
arr.reduce(
(grouped, obj) => ({
...grouped,
[groupFn(obj)]: [
...(grouped[groupFn(obj)] || []),
obj,
],
}),
{}
);
const fruits = [
{ name: 'pineapple🍍', color: '🟡' },
{ name: 'apple🍎', color: '🔴' },
{ name: 'banana🍌', color: '🟡' },
{ name: 'strawberry🍓', color: '🔴' },
];
const groupedByNameLength = groupBy(
fruits,
(fruit) => fruit.color
);
console.log(groupedByNameLength);
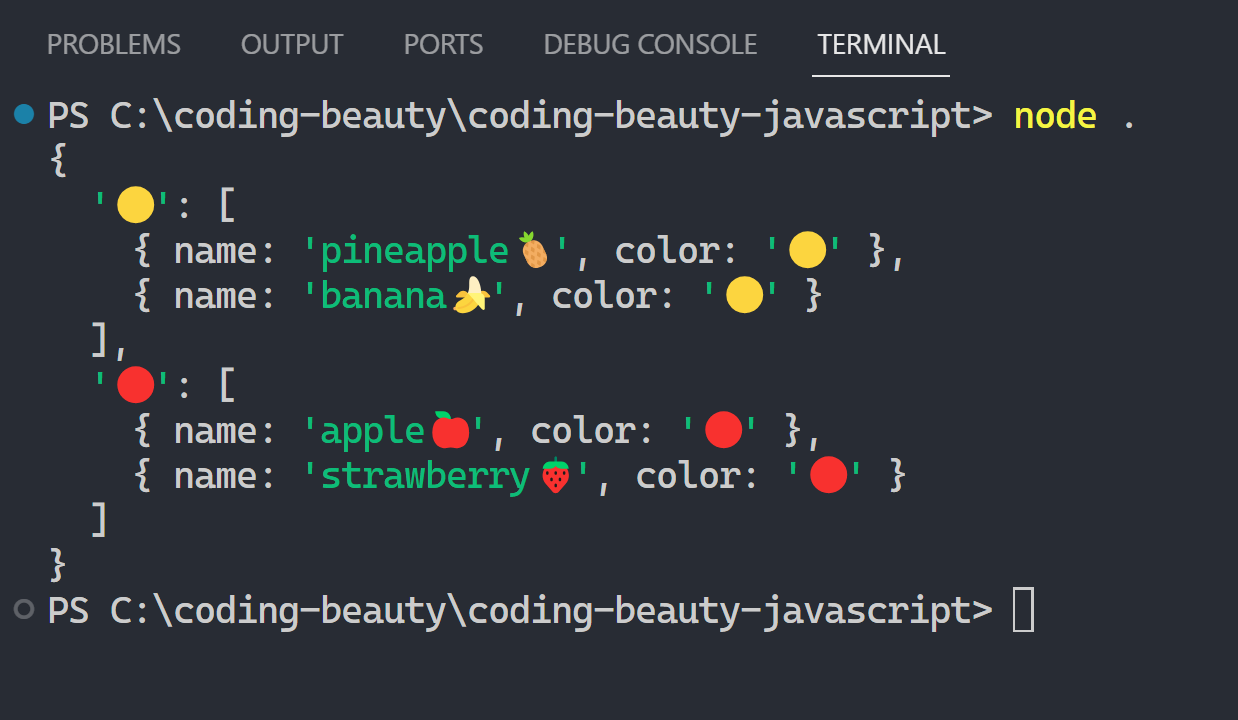
3. An ID for everyone
Perfection.
const generateRandomUUID = (a) =>
a
? (a ^ ((Math.random() * 16) >> (a / 4))).toString(16)
: ([1e7] + -1e3 + -4e3 + -8e3 + -1e11).replace(
/[018]/g,
generateRandomUUID
);
console.log(generateRandomUUID()); // f138f635-acbd-4f78-9be5-ca3198c4cf34
console.log(generateRandomUUID()); // 8935bb0d-6503-441f-bb25-7bc685b5b5bc
Just look at the language concepts working together here; there’s basic arithmetic, powers, random values, methods, bitshifting, regexes, callback functions, recursion, exponentiation… it’s perfection.
4. Flipping the string script: Reverse a string
Not this✖️
const reverseString = (str) => {
let reversed = '';
for (let i = str.length - 1; i >= 0; i--) {
const ch = str[i];
reversed += ch;
}
return reversed;
};
const reverse = reverseString('Indestructible!');
console.log(reverse); // !elbitcurtsednI
But this✅:
const reverseString = (str) => str.split('').reverse().join('');
const reverse = reverseString('Indestructible!');
console.log(reverse); // !elbitcurtsednI
5. Painting with code: Generate random hex color
1 line to generate a random hex color:
const randomHexColor = () =>
`#${Math.random().toString(16).slice(2, 8).padEnd(6, '0')}`;
console.log(randomHexColor()); // #7a10ba (varies)
console.log(randomHexColor()); // #65abdc (varies)
6. Array equality
Check array equality with a one-liner…
Not this✖️:
const areEqual = (arr1, arr2) => {
if (arr1.length === arr2.length) {
for (const num of arr1) {
if (!arr2.includes(num)) {
return false;
}
}
return true;
}
return false;
};
But this✅:
const areEqual = (arr1, arr2) =>
arr1.sort().join(',') === arr2.sort().join(',');
Or✅:
// For more complex objects
// and sort() will probably have a defined callback
const areEqual = (arr1, arr2) =>
JSON.stringify(arr1.sort()) === JSON.stringify(arr2.sort());
7. Clean and clutter-free arrays
Shortest way to remove duplicates from an array?
Not this✖️:
const removeDuplicates = (arr) => {
const result = [];
for (const num of arr) {
if (!result.includes(num)) {
result.push(num);
}
}
return result;
};
const arr = [1, 2, 3, 4, 5, 3, 1, 2, 5];
const distinct = removeDuplicates(arr);
console.log(distinct); // [1, 2, 3, 4, 5]
But this✅:
const arr = [1, 2, 3, 4, 5, 3, 1, 2, 5];
const distinct = [...new Set(arr)];
console.log(distinct); // [1, 2, 3, 4, 5]
These used to be like the only reason anyone ever cared for Sets — until we got these 7 amazing new methods.
8. That’s not an a email: Validation
Email validation one-liners are all about that regex:
const isValidEmail = (email) =>
/^[\w-]+(\.[\w-]+)*@([\w-]+\.)+[a-zA-Z]{2,7}$/.test(
email
);
console.log(isValidEmail('[email protected]')); // true
console.log(isValidEmail('[email protected]')); // false
console.log(isValidEmail('codingbeautydev.com')); // false
console.log(isValidEmail('hi@')); // false
console.log(isValidEmail('hi@codingbeautydev&12')); // false
But I’ve seen monstrosities like this! 👇
const isValidEmail = (email) =>
/^(([^<>()[\]\\.,;:\s@\"]+(\.[^<>()[\]\\.,;:\s@\"]+)*)|(\".+\"))@((\[[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}\])|(([a-zA-Z\-0-9]+\.)+[a-zA-Z]{2,}))$/.test(
email
);
console.log(isValidEmail('[email protected]')); // true
console.log(isValidEmail('[email protected]')); // false
console.log(isValidEmail('codingbeautydev.com')); // false
console.log(isValidEmail('hi@')); // false
console.log(isValidEmail('hi@codingbeautydev&12')); // false
And even these 😮:
// ❗❗
const isValidEmail = (email) =>
/(?:[a-z0-9!#$%&'*+/=?^_`{|}~-]+(?:\.[a-z0-9!#$%&'*+/=?^_`{|}~-]+)*|"(?:[\x01-\x08\x0b\x0c\x0e-\x1f\x21\x23-\x5b\x5d-\x7f]|\\[\x01-\x09\x0b\x0c\x0e-\x7f])*")@(?:(?:[a-z0-9](?:[a-z0-9-]*[a-z0-9])?\.)+[a-z0-9](?:[a-z0-9-]*[a-z0-9])?|\[(?:(?:25[0-5]|2[0-4][0-9]|[01]?[0-9][0-9]?)\.){3}(?:25[0-5]|2[0-4][0-9]|[01]?[0-9][0-9]?|[a-z0-9-]*[a-z0-9]:(?:[\x01-\x08\x0b\x0c\x0e-\x1f\x21-\x5a\x53-\x7f]|\\[\x01-\x09\x0b\x0c\x0e-\x7f])+)\])/.test(
email
);
console.log(isValidEmail('[email protected]')); // true
console.log(isValidEmail('[email protected]')); // false
console.log(isValidEmail('codingbeautydev.com')); // false
console.log(isValidEmail('hi@')); // false
console.log(isValidEmail('hi@codingbeautydev&12')); // false
It’s probably about being as thorough as possible – let’s say the 1st one catches like 95% of wrong emails, then the 2nd like 99%.
The last one here is actually the official RFC 2822 standard for validating emails – so we’re looking at 100% coverage.
9. Data translation: JSON to Maps
const jsonToMap = (json) => new Map(Object.entries(JSON.parse(json)));
const json = '{"user1":"John","user2":"Kate","user3":"Peter"}';
const map = jsonToMap(json);
// Kate
console.log(map.get('user2'));
// Map(3) { 'user1' => 'John', 'user2' => 'Kate', 'user3' => 'Peter' }
console.log(map);
10. Slithering snake to calm camel
Easily convert from snake casing to camel casing with zero temporary variables.
const snakeToCamelCase = (s) =>
s
.toLowerCase()
.replace(/(_\w)/g, (w) => w.toUpperCase().substr(1));
const str1 = 'passion_growth';
const str2 = 'coding_beauty';
console.log(snakeToCamelCase(str1)); // passionGrowth
console.log(snakeToCamelCase(str2)); // codingBeauty
Final thoughts
Countless operations jam-packed into a single statement; A race from input start to output finish with no breaks, a free flow of high-level processing. A testament of coding ability and mastery.
This is the power and beauty of JavaScript one-liners.
Every Crazy Thing JavaScript Does
A captivating guide to the subtle caveats and lesser-known parts of JavaScript.
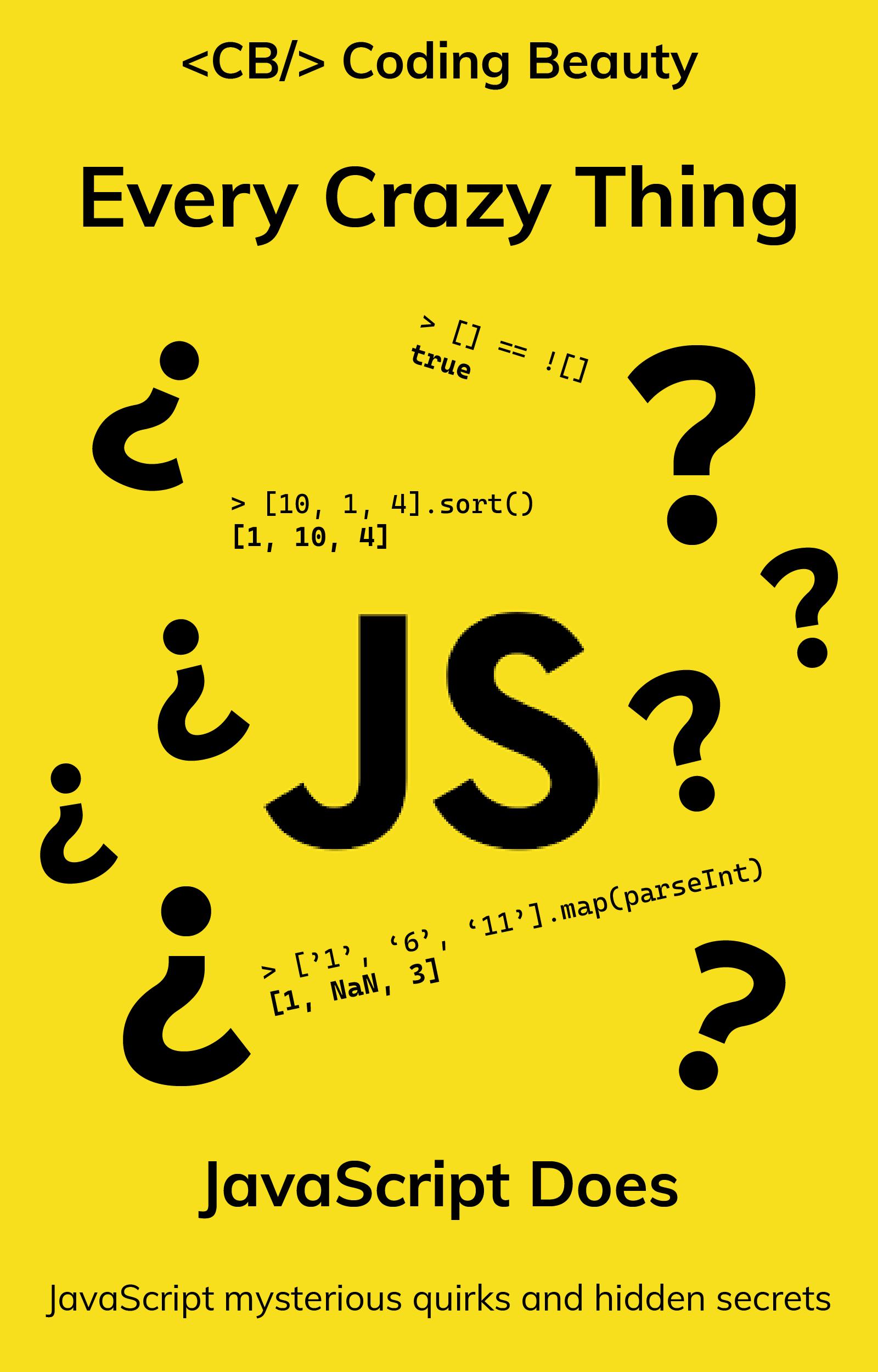
Example 8 ‘Check if Two Arrays Contain the Same Values’ is incorrect. Please check the test cases below.
“`javascript
const areEqual = (arr1, arr2) =>
arr1.length === arr2.length && arr1.every((num) => arr2.includes(num));
// Arrays have different items.
const arrA = [1, 1, 3, 4];
const arrB = [1, 2, 3, 4];
console.log(areEqual(arrA, arrB)); // true
// Arrays have the same items. But the elements occur a different number of times.
const arr1 = [1, 1, 2, 3, 4];
const arr2 = [1, 2, 2, 3, 4];
console.log(areEqual(arr1, arr2)); // true
“`
You’re right Victor, I have updated the example with a better one-liner.👍